Create a Lightning-Fast AI Image Generator in 5 Minutes with Fal AI and Next.js
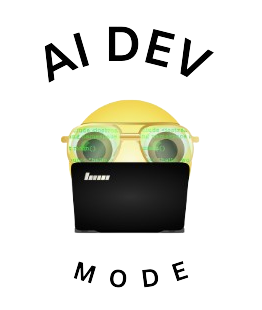
Create a Lightning-Fast AI Image Generator in 5 Minutes with Fal AI and Next.js
In this tutorial, we'll build a simple yet powerful AI image generator using Fal AI and Next.js with the App Router. By the end, you'll have a functional web application that can generate images based on text prompts.
Prerequisites
- Basic knowledge of React and Next.js
- Node.js and npm installed on your machine
- A Fal AI account and API key (sign up at fal.ai)
Step 1: Set Up Your Next.js Project
First, let's create a new Next.js project with the App Router:
npx create-next-app@latest fal-ai-image-generator
cd fal-ai-image-generator
When prompted, choose the following options:
- Use TypeScript: Yes
- Use ESLint: Yes
- Use Tailwind CSS: Yes
- Use src/ directory: No
- Use App Router: Yes
- Customize default import alias: No
Step 2: Install Dependencies
Install the necessary Fal AI libraries:
npm install @fal-ai/serverless-client @fal-ai/serverless-proxy
Step 3: Set Up Fal AI Proxy
Create a new file at app/api/fal/proxy/route.ts
and add the following code:
import { route } from "@fal-ai/serverless-proxy/nextjs";
export const { GET, POST } = route;
This sets up a proxy to securely handle Fal AI requests.
Step 4: Configure Fal AI Client
Update your app/layout.tsx
file to include the Fal AI client configuration:
import * as fal from "@fal-ai/serverless-client";
import { Inter } from "next/font/google";
import "./globals.css";
const inter = Inter({ subsets: ["latin"] });
fal.config({
proxyUrl: "/api/fal/proxy",
});
export const metadata = {
title: "Fal AI Image Generator",
description: "Generate images with AI",
};
export default function RootLayout({
children,
}: {
children: React.ReactNode;
}) {
return (
<html lang="en">
<body className={inter.className}>{children}</body>
</html>
);
}
Step 5: Create the Image Generator Component
Create a new file at app/components/ImageGenerator.tsx
and add the following code:
import React, { useState } from "react";
import * as fal from "@fal-ai/serverless-client";
const ImageGenerator = () => {
const [prompt, setPrompt] = useState("");
const [generatedImage, setGeneratedImage] = useState("");
const [isGenerating, setIsGenerating] = useState(false);
const [error, setError] = useState("");
const handleGenerate = async (e: React.FormEvent) => {
e.preventDefault();
if (!prompt) return;
setIsGenerating(true);
setError("");
try {
const result: any = await fal.subscribe("fal-ai/flux/schnell", {
input: {
prompt,
},
logs: true,
onQueueUpdate: (update) => {
if (update.status === "IN_PROGRESS") {
console.log(update.logs.map((log) => log.message));
}
},
});
if (result.images && result.images[0]) {
setGeneratedImage(result.images[0].url);
} else {
setError("No image was generated");
}
} catch (error: any) {
console.error("Error generating image:", error);
setError(`An error occurred: ${error.message}`);
} finally {
setIsGenerating(false);
}
};
return (
<div className="w-full mx-auto p-4 bg-white rounded-md shadow-md">
<form onSubmit={handleGenerate} className="space-y-4">
<textarea
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
placeholder="Enter your image prompt here..."
className="w-full p-2 border rounded-md"
rows={4}
/>
<button
type="submit"
disabled={isGenerating}
className="w-full bg-blue-500 text-white p-2 rounded-md hover:bg-blue-600 disabled:bg-gray-400"
>
{isGenerating ? "Generating..." : "Generate Image"}
</button>
</form>
{error && <p className="text-red-500 mt-4">{error}</p>}
{generatedImage && (
<div className="mt-6">
<img
src={generatedImage}
alt="Generated image"
className="rounded-md shadow-lg max-w-full h-auto"
/>
</div>
)}
</div>
);
};
export default ImageGenerator;
Step 6: Update the Home Page
Replace the content of app/page.tsx
with the following:
import ImageGenerator from "./components/ImageGenerator";
export default function Home() {
return (
<main className="flex min-h-screen flex-col items-center justify-between p-24">
<div className="z-10 max-w-5xl w-full items-center justify-between font-mono text-sm">
<h1 className="text-4xl font-bold mb-8 text-center">
AI Image Generator
</h1>
<ImageGenerator />
</div>
</main>
);
}
Step 7: Set Up Environment Variables
Create a .env.local
file in the root of your project and add your Fal AI API key:
FAL_KEY=your_fal_ai_api_key_here
Replace your_fal_ai_api_key_here
with your actual Fal AI API key.
Step 8: Run Your Application
Start your Next.js development server:
npm run dev
Visit http://localhost:3000
in your browser, and you should see your AI image generator in action!
Conclusion
Congratulations! You've just built a simple AI image generator using Fal AI and Next.js. This application demonstrates the power of AI in creating images from text prompts. Feel free to expand on this project by adding features like image downloading, different AI models, or more styling options.
Remember to handle your API keys securely and never expose them in client-side code.
More Info: https://fal.ai/docs