PDF Processing with Gemini: From Documents to Structured Data
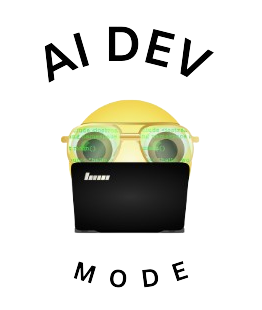
Processing PDFs has traditionally been a complex task requiring multiple tools and complex pipelines. With Google's Gemini 1.5 and the Vercel AI SDK, we can simplify this process significantly while getting structured outputs using Zod. Let's explore how to build a fast and modern PDF processing application.
The Challenge
PDF analysis has traditionally been a headache for developers. Whether you're trying to extract information from research papers, contracts, or technical documentation, the existing solutions often feel like overkill for simple tasks.
Most developers end up either:
- Writing complex regex patterns to parse text
- Paying for expensive document analysis APIs
- Building elaborate ML pipelines
- Managing multiple libraries for different document types
With Gemini's multimodal capabilities combined with Zod for type-safe outputs in Node.js, we can turn complex document analysis into a few lines of code.
Setting Up the Project
First, install the required packages:
npm init -y
npm install ai @ai-sdk/google zod
Before running the code, make sure to get your API key:
- Visit Google AI Studio
- Create a new API key
- Add it to your environment:
export GOOGLE_GENERATIVE_AI_API_KEY=your-api-key-here
Or create a .env
file:
GOOGLE_GENERATIVE_AI_API_KEY=your-api-key-here
Complete Code
Here's all you need:
async function main() {
try {
const response = await generateObject({
model: google("gemini-1.5-flash-latest", {
structuredOutputs: false,
}),
schema: Summary,
messages: [
{
role: "user",
content: [
{
type: "text",
text: "Provide a summary and main topics from this document",
},
{
type: "file",
data: fs.readFileSync("./gemini.pdf"),
mimeType: "application/pdf",
},
],
},
],
});
const result = response.object;
console.log("Summary:", result.summary);
console.log("\nMain Topics:");
result.mainTopics.forEach((topic, index) => {
console.log(`${index + 1}. ${topic}`);
});
} catch (error) {
console.error("Error processing PDF:", error);
}
}
main().catch(console.error);
Run with:
node index.js
Example Output
Summary: This paper introduces the Gemini 1.5 family of models, which are highly compute-efficient multimodal models capable of recalling and reasoning over fine-grained information from millions of tokens of context.
The family includes two new models: Gemini 1.5 Pro and Gemini 1.5 Flash. Gemini 1.5 Pro outperforms the February version on the great majority of capabilities and benchmarks, while Gemini 1.5 Flash is a more lightweight variant designed for efficiency with minimal regression in quality.
Gemini 1.5 models achieve near-perfect recall on long-context retrieval tasks across modalities, improve the state-of-the-art in long-document QA, long-video QA and long-context ASR, and match or surpass Gemini 1.0 Ultra’s state-of-the-art performance across a broad set of benchmarks.
Studying the limits of Gemini 1.5’s long-context ability, we find continued improvement in next-token prediction and near-perfect retrieval (>99%) up to at least 10M tokens, a generational leap over existing models such as Claude 3.0 (200k) and GPT-4 Turbo (128k).
Finally, we highlight real-world use cases, such as Gemini 1.5 collaborating with professionals on completing their tasks achieving 26 to 75% time savings across 10 different job categories, as well as surprising new capabilities of large language models at the frontier; when given a grammar manual for Kalamang, a language with fewer than 200 speakers worldwide, the model learns to translate English to Kalamang at a similar level to a person who learned from the same content.
Main Topics:
1. multimodal understanding
2. long-context reasoning
3. efficiency
4. multi-lingual capabilities
5. safety
6. security
7. responsibility
Why This Works
Our approach brings several advantages:
- Zero Setup - No configuration files or complex installations
- Type Safety - Zod ensures your data matches expected formats
- Multimodal Support - Works with PDFs, images, video, and audio
- Instant Results - Direct PDF to structured data conversion
- Future-Proof - As Gemini improves, your code stays the same
What's Next
Start experimenting with different document types and schemas. The same code works for analyzing:
- Technical documentation
- Research papers
- Business reports
- Legal documents
Conclusion
With Gemini 1.5 and Next.js, we've transformed complex PDF processing into a simple, type-safe application. This approach eliminates the need for complex document processing pipelines while providing structured outputs that are easy to work with.Remember to check the Vercel AI SDK documentation for updates and new features as they become available.
Framework Integration
This simple PDF processing can be integrated into any modern framework - React, Typescript, Next.js, Vue, Svelte, and more.
📫 DM Me for consulting inquiries and professional work.